Posts on this site are never sponsored.
This was the first php script that I ever looked up sooooo many years ago. It’s really quite simple. You want to show visitors a countdown in days to a certain event in the future. Since PHP can provide the current time on the server to the very second, simply calculate the desired date (in seconds) and subtract from the server date. More complicated scripts can give a choice between hours, months, or days remaining, can compensate for a server time different than your current time, and can probably dress up the output a bit better.
I’ve spiced this code up a bit by making it a function (thus enhancing reusability), but otherwise, let’s go with simple, shall we?
Here’s the code:
<?php
function countdown( $event, $month, $day, $year )
{
// subtract desired date from current date and give an answer in terms of days
$remain = ceil( ( mktime( 0,0,0,$month,$day,$year ) - time() ) / 86400 );
// show the number of days left
if( $remain > 0 )
{
print "<p><strong>$remain</strong> more sleeps until $event</p>";
}
// if the event has arrived, say so!
else
{
print "<p>$event has arrived!</p>";
}
}
// call the function
countdown( "Christmas", 12, 25, 2025 );
?>
How about some more fun:
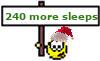
Update: I have since made a countdown plugin for WordPress that incorporates the basic concept.
Posted in Web development tutorials | 11 Comments »
Contrary to what many people believe, you do not need to shell out hundreds of dollars to create PDFs. There are many benefits to having the full-featured Adobe Acrobat (such as the ability to edit a pdf) but if you’re just looking to create a PDF of your school report in Word, you can download a free PDF convertor such as CutePDF. Do a quick Google search for free PDF makers and you’ll find many others.
So what are you waiting for? Go explore the world of free PDF-ing!
And heck, Adobe Acrobat can’t even do things like convert to grayscale.
Posted in Computer Stuff | 4 Comments »
First of all, let me state that with all of the sites that I mention, unless they are my own silly creations, I am receiving nothing for the free plugs.

Anyway, so the great news from Skype earlier this year was that it was offering free PC-to-phone calls within North America until the end of the year. However, this rather new site called Jajah takes the whole PC/phone convenience thing one step further. You need your computer only to initiate the call. It works like this:
1) Register your phone number
2) Enter the number you wish to call on the Jajah website
3) Your phone rings (not your computer) and you pick up
4) Your friend’s phone rings
Boom — phone-to-phone calls, initiated at the Jajah website. Check their Free Calls FAQ Page to make sure they’re not messing with us. They do state, however, that there really is “no catch”. I’m interested to see what will become of this Jajah…
Also, make sure you check the rate page to make sure that your call is free (remember, it’s between many countries that it’s free, but not all!).
————————-
August 8th, 2006 update: so, what’s up with this… yet another startup offering a free PC-to-phone service (although not phone-to-phone like Jajah). They call themselves Gizmo, and work very similarly to Skype.
————————-
January 28th, 2007 update: a few months on Jajah, a few more friends convinced, many dollars saved! Just make sure to keep your account “active” by making a free call at least once a week!
Posted in Computer Stuff, Consumerism, Telephony | 23 Comments »
Sick of the Magic 8-ball? Or do you simply not have one? Get The Blog to answer all of your trivial (or not so trivial) yes/no questions!
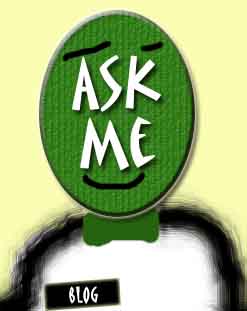
Now, you might say, this is more “useless” than “useful”. However, consider this scenario: Trevor cannot decide whether he should eat a sub for lunch. Compared to all of the other problems that this world is presented with (wars, starvation, etc.), Trevor really ought not waste too much time on such a trivial matter. Therefore, by getting The Blog to offer its opinion quickly on the lunch issue, Trevor can then get on with saving the world. I would say that’s some darn useful crap.
Posted in Tomfoolery | 2 Comments »
So there I was typing in every possible keyword combination into Google about “resizing text boxes without resizing the text itself” (and 200 other variations over the course of an hour). All I wanted to do was to change text from being left-aligned to being centered, but the text box was obviously the wrong size to be centered. Naturally, I went to resize the text box. But when I resized the text box, the text itself was changed as well!
From:
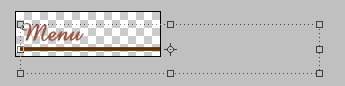
To:
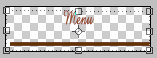
Trying to tweak every conceivable Photoshop option and using ctrl+click, shift+right-click, scream-at-computer-click, etc. got me nowhere. So thanks for nothing to Google and thanks for nothing to my brain.
Yeah, I could have just created a new text box, but that would have meant giving in. So, after an hour I sent the file to Derek (smart guy) and complained. He replied: “I don’t have the problem — I can resize the text box and the font size isn’t changed.”
What!?!? Is this a joke!?!? I went back to Photoshop and… ah… for some reason a light finally turned on: I should have simply resized while using the Text Tool; all along, I was trying to use the arrow to resize the text box.

Thankfully, somebody else has had this problem (way back in 2003, and his question in a newsgroup was actually never answered), so before I go and bang my head against the wall, maybe I can blame Adobe Photoshop for poor design? OK fine, I’m an idiot. But now I am writing about this in the hopes that someone, somewhere, sometime, will have this same problem and will find this post. And that hour that they will save (well, probably less since I’m a bit slow) is priceless.
Posted in Computer Stuff | 112 Comments »